I’m currently working on a installer. Meanwhile, here’s the long way of connecting the xArm/LeArm via USB to Ubuntu.
If you have any trouble with the following or have any questions, reach out to my in the xArm Enthusiasts group on Facebook. If reporting a problem, please provide the error output.
Install Visual Studio Code and the Python Extension
In this article I will be using Visual Studio Code. You can, of course, use any IDE you wish, however, you may need to translate or ignore some of the following steps.
To install VSCode on Ubuntu:
- Click the Ubuntu Software button (
).
- Search (
) in upper-left corner of window) for “VSCode”.
- Select Visual Studio Code from the list.
- Click the Install button.
Check and Install Python and PIP
To check if Python is installed, run the following command in Terminal. It should return a version number.
$ python3 --version
If command not found, install Python by running the following command in Terminal.
sudo apt install python3
To check if PIP is installed, run the following command in Terminal. It should return a version number.
pip3 --version
If command not found, install PIP by running the following command in Terminal.
sudo apt install python3-pip
Run VSCode and set up virtual Environment
From Visual Studio Code, open folder for your project. Click File in the header menu, then click Open Folder.
Navigate folders and create folders as needed until you are at your prefered project path and click OK.
While we have VSCode open, install the Microsoft Python extension. Search for and install ms-python.python
If the VSCode terminal is not already open, open it by either pressing Ctrl-` (backtick) or by Clicking Terminal in the header menu and then New Terminal.
Creating virtual environment requires software python3-venv. In the VSCode terminal, run the following command. It is not absolutely necessary to create a virtual environment but there are several benefits to doing so including sandboxing your loaded modules and building a requirements list.
$ python3 -m venv env
If you receive a “No module found” error, install venv by running the follow command in the VSCode terminal. Run the command above again.
$ sudo apt install python3-venv
Start virtual environment by running the following command in the VSCode terminal.
$ source env/bin/activate
You should see “(env)” preceding the command prompt.
Click Run and Debug and create a launch json file from the link under the Debug button. Choose Python as your interpreter.
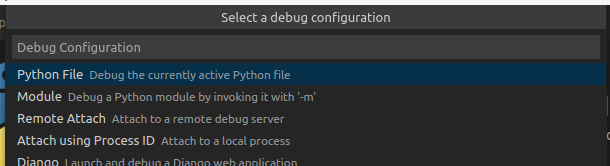
Install HidApi
Install python-hidapi. To learn more about this module, visit https://github.com/trezor/cython-hidapi.
Run the following commands to install prerequisite libraries from the VSCode terminal.
$ sudo apt install python3-dev libusb-1.0-0-dev libudev-dev
$ pip3 install --upgrade setuptools
$ pip3 install hidapi
Ubuntu and Lubuntu to not register unknown USB device types so we’ll have to inform the system to allow the xArm/LeArm.
From the VSCode terminal or Ubuntu’s terminal, run the following command.
$ sudo nano /etc/udev/rules.d/99-myrules.rules
Add the following line to the file.
SUBSYSTEM=="usb", ATTR{idVendor}=="0483", ATTR{idProduct}=="5750", MODE="0660", GROUP="plugdev"
Save the file and exit Nano. Ctrl-W and then Ctrl-X.
Run the following command to activate the new rules.
$ sudo udevadm control --reload-rules
Congratulations! That may have seemed like a lot of setup but the hard part is done. Now let’s run some test code.
Copy the following code into a py file in your project and run it.
import hid
import time
def pad_or_truncate(some_list, target_len):
return some_list[:target_len] + [0]*(target_len - len(some_list))
dev = hid.device()
dev.open(0x0483, 0x5750)
print("Manufacturer: %s" % dev.get_manufacturer_string())
print("Product: %s" % dev.get_product_string())
print("Serial No: %s" % dev.get_serial_number_string())
# enable non-blocking mode
dev.set_nonblocking(1)
# write some data to the device
print("Write the data")
data = [0, 0x55, 0x55, 2, 0x0f]
data = pad_or_truncate(data, 65)
dev.write(data)
# wait
#time.sleep(0.05)
# read back the answer
print("Read the data")
response = []
while True:
d = dev.read(64, 50)
if d:
response.extend(d)
else:
break
print(f"{(response[5] * 256 + response[4])/1000} VDC")
print("Closing the device")
dev.close()